What is Device-Binding?
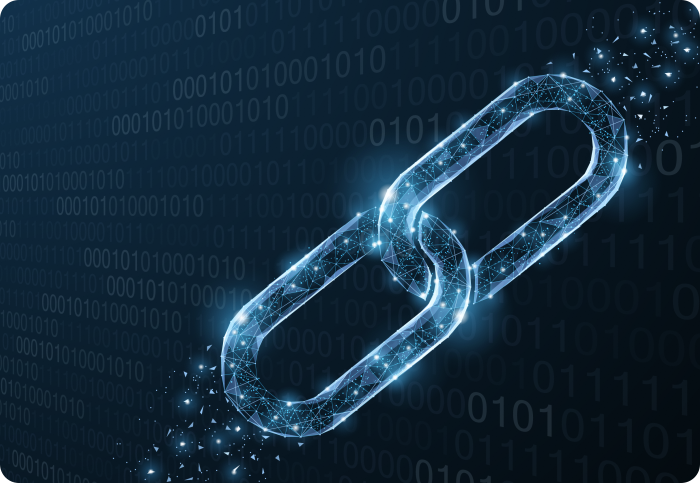
If a device is programmed to interact with the LaRoomy App, everybody with the LaRoomy App installed is able to connect to this device. But what if you want an exclusive connection to your device? This is realized with the Device-Binding.
How it works
When a user enables the Device-Binding by pressing the appropriate button on the Device-Settings-Page, a random Binding-Key is generated and transmitted to the remote device. The device is responsible for storing the key permanently and to authenticate the app in a connection process. Only if the app with the right key is connecting, the device should grant access to it's Device-Properties and their functionality.
Ways to implement Device-Binding
The most comfortable way is to enable the internal Device-Binding-Handler:
LaRoomyApi .enableInternalBindingHandler(true);
The LaRoomy Api takes the responsibility of the the complete binding-process. In this case the binding callback event is not called. If there is the need to implement some custom behaviour on Binding-Events, the internal handler must remain disabled. In this case the DeviceBindingController can be used to implement the DeviceBinding:
// define the callback for the remote app-user events
class RemoteEvents : public ILaroomyAppCallback
{
// handle binding transmissions
BindingResponseType onBindingTransmissionReceived(BindingTransmissionTypes bType , const String &key ) override
{
/*
do some custom processing here
*/
return DeviceBindingController .handleBindingTransmission(bType , key );
}
};
void setup()
{
// put your setup code here, to run once:
/*
other setup ...
*/
// set the callback handler for remote events
LaRoomyApi .setCallbackInterface(
dynamic_cast<ILaroomyAppCallback *>(
new RemoteEvents()));
// load the required parameter from permanent storage and set it
LaRoomyApi .setDeviceBindingAuthenticationRequired(
DeviceBindingController .isBindingRequired()
);
/* other setup ... */
}
The last way to implement Device-Binding is to manually implement all logic to handle Binding transmissions and key storage. If this is done there are some important points to consider:
1. The callback-method is invoked on three cases:
- Binding is enabled by the user - The transmitted Binding-Key must be saved and a binding required parameter must be saved.
- Binding is released by the user - The binding required parameter must be cleared.
- An authentication request is done during the connection process - The transmitted key must be compared and the result must be indicated with the return value of the callback method.
All cases must be handled and the appropriate value must be returned. (BindingResponseType)
2. When the device is started, the saved required paramter must be loaded and set to the LaRoomy Api:
void setup()
{
// put your setup code here, to run once:
// load the required parameter from permanent storage
auto bRequired = ..
/*
other setup ...
*/
LaRoomyApi .setDeviceBindingAuthenticationRequired(bRequired );
/* other setup ... */
}